Monday, July 9, 2012
Forgot password script in PHP - Step by Step Tutorial!
Monday, July 9, 2012 by v
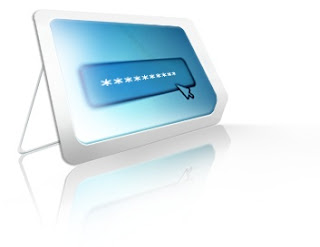
Step 1: In this very first step, I assume that you have wamp server installed on your computer. If you do not have it installed please refer this article for details.
Step 2: Next thing you should have is a user table. I have written the create table code below. Copy it and execute it under SQL section of PhpMyAdmin.
----------------------------------------
CREATE TABLE `users` (
`id` bigint(20) NOT NULL auto_increment,
`md5_id` varchar(200) collate latin1_general_ci NOT NULL default '',
`full_name` tinytext collate latin1_general_ci NOT NULL,
`user_name` varchar(200) collate latin1_general_ci NOT NULL default '',
`user_email` varchar(220) collate latin1_general_ci NOT NULL default '',
`pwd` varchar(220) collate latin1_general_ci NOT NULL default '',
`activation_code` int(10) NOT NULL default '0',
PRIMARY KEY (`id`),
UNIQUE KEY `user_email` (`user_email`),
FULLTEXT KEY `idx_search` (`full_name`,`user_email`,`user_name`)
) ENGINE=MyISAM DEFAULT CHARSET=latin1 COLLATE=latin1_general_ci AUTO_INCREMENT=55 ;
----------------------------------------
Step 3: Once we are done with the table, let's create a file called "forgot.php".
Step 4: Write following lines of code in it to get a simple text box with a submit button.
----------------------------------------
<form action="forgot.php" method="post">
Enter you email ID: <input type="text" name="email">
<input type="submit" name="submit" value="Send">
</form>
----------------------------------------
Step 5: Save it as forgot.php and run it on your browser by pointing to localhost. It should look something like this:
Step 6: So, you can see above form in your browser? Great! Let's move ahead! It's time to make this submit button and text box work. For this, we first need to make sure that e-mail entered in the text box does exist in database. If it does not, then script must raise an error. Below is the code (Make sure that you have set correct parameters for connection)
----------------------------------------
<?php error_reporting(0);
$email=$_POST['email'];
if($_POST['submit']=='Send')
{
mysql_connect('localhost','root','') or die(mysql_error);
mysql_select_db('test');
$query="select * from users where user_email='$email'";
$result=mysql_query($query) or die(error);
if(mysql_num_rows($result))
{
echo "User exist";
}
else
{
echo "No user exist with this email id";
}
}
?>
<form action="forgot.php" method="post">
Enter you email ID: <input type="text" name="email">
<input type="submit" name="submit" value="Send">
</form>
----------------------------------------
Step 7: Brief explaination of above code goes like this:Function "mysql_connect()" will connect to the database. Here we have passed default parameters for 'Host', 'User' and 'Password'.
Function "mysql_select_db()" select the database name from the PhpMyAdmin. Here we have chosen database name "test". If it could not select the database due to some errors, it will raise an error and print the content written in die(), ('Error' in our case).
Finally, $query is a variable which stores the query string, $result variable will capture the outcomes when query is executed using inbuilt function mysql_query().
After all, we have to put a simple check for email existence confirmation. Normally, we have to check whether there is any row exist in database which the provided email or not. So, here is another cool PHP function to test that, called "mysql_num_rows()". You can check the code above integrated with if...end if statement.
Step 8: Our next step would be to add code to send an confirmation email when email entered in text box, does exist in database. For that, we will be making use of another simple mail function.
Its syntax is like this:
----------------------------------------
mail(to,subject,message,headers,parameters)----------------------------------------
So, we will send an email to the provided email using mail function. Below is code which is needed when email exist.----------------------------------------
if(mysql_num_rows($result))
{
$code=rand(100,999);
$message="You activation link is: http://yourwebsitename.com/forgot.php?email=$email&code=$code
mail($email, "Subject Goes Here", $message);
echo "Email sent";
}
else
{
echo "No user exist with this email id";
}
----------------------------------------
Step 9: Above code is modification to existing code with new mail function in it. Replace "yourwebsitename" with the address to your "forgot.php" page to get the script working. Moreover, also update the activation code in the database filed "activation_code", it's necessary.
Step 10: Run the script, you should get an rest password mail. Click on the link in email which will redirect you back to forgot.php on your website with URL parameters as email and code filled. So, you have to fetch these two values once again and compare the code with the one present in database with that particular email. If it exist, continue.
Step 11: Now, we will have a field for new password and a submit button. Because everything is verified, we can set up an update command to the password field after user enter a new password and hit enter.
It is similar to the above process of making email form with little variations and I believe that now it would be easy to achieve it to for you. Please do work and let me know in case you are facing any bug.
Subscribe to:
Post Comments (Atom)
0 Responses to “Forgot password script in PHP - Step by Step Tutorial!”
Post a Comment