Tuesday, April 24, 2012
Instant username validation using jQuery in PHP
Tuesday, April 24, 2012 by v
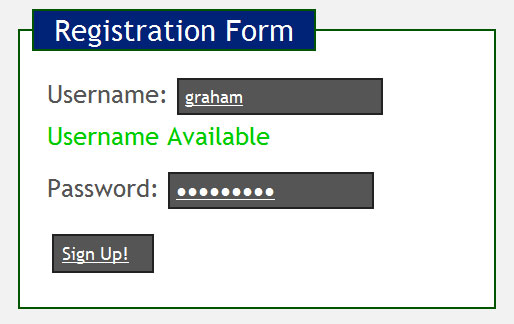
While working on any php script which includes validation measures, the reliability and usability of the software becomes the priority for developer. It can be achieved through various aspects such as by keeping simple design and giving as much required feedback to user as much possible. In this article, we will see one minor section of such usability features which can be used in registration form to make program more user-friendly. It is about instant username checking or validating as soon as user type in the username. For this, we also need jQuery which will execute the query each time we enter a character in the username field. Overall, script is very much easy to use and implement in any type of website with any design.
Steps to create a username validation script in PHP:
Step 1: In this very first step, we will create a database with the name "databaseCheck" in MySQL. Of course, for simplicity, you can prefer phpmyadmin for it. You can install WampServer for phpmyadmin.
Step 2: For this example purpose, we are keeping only one field in the database which is named "username". Thats it. You can add 3-4 user names in it. Let us add 'Mekey', 'Salaria', 'user', 'admin'.
Step 3: Now, as you are ready with your database, its time to write some PHP code. We will go step by step for each single file.
Step 4: Create a PHP with name "index.php" and add the following code in it. I have also written description of code in the form of comments within the code given below to make it more clear to you.
<html>
<head>
<title>Instant Username Validation script by MyNameIs</title>
</head>
<script src="http://ajax.googleapis.com/ajax/libs/jquery/1.3.2/jquery.js" type="text/javascript">
</script>
<?php
/*This part of script is very important. It will check for the username in the database, if the username
is found in database then it will show an error image if not, then user available tick mark will be shown. Javascript
calls check.php in order to check the username value.
Also, some CSS part is embedded within the javascript below but its only for designing purpose which has nothing to do with
functioning of script
*/
?>
<script>
$(document).ready(function(){
$('#username').keyup(username_check);
});
function username_check(){
var username = $('#username').val();
if(username == "" || username.length < 4){
$('#username').css('border', '3px #CCC solid');
$('#tick').hide();
}else{
jQuery.ajax({
type: "POST",
url: "check.php",
data: 'username='+ username,
cache: false,
success: function(response){
if(response == 1){
$('#username').css('border', '3px #C33 solid');
$('#tick').hide();
$('#cross').fadeIn();
}else{
$('#username').css('border', '3px #090 solid');
$('#cross').hide();
$('#tick').fadeIn();
}
}
});
}
}
</script>
<style>
#username{
padding:3px;
font-size:18px;
border:3px #CCC solid;
}
#tick{display:none}
#cross{display:none}
</style>
</head>
<body>
Here are some usernames that have been put in the database:<br/><br/>
Mekey, Salaria, user, admin<br/><br/>
Username: <input name="username" id="username" type="text" />
<img id="tick" src="tick.png" width="16" height="16"/>
<img id="cross" src="cross.png" width="16" height="16"/>
</body>
</html>
Step 5: After completely understanding index.php you are ready for the next part. You can see in above script that JavaScript has called another PHP file named "check.php" with the method POST. So, it send all of the grabbed information, i.e., username, to the check.php, where it validates the information. This check.php is connected to the database using another file called "dbConnector.php".
Here is the code to use in this file:
<?php
class DbConnector {
var $theQuery;
var $link;
function DbConnector(){
// Get the main settings from the array we just loaded
$host = 'HOST'; //it use to be localhost in most of cases
$db = 'DATABASE'; //set the name of database in which you have created table userCheck
$user = 'USERNAME'; //here you need to write username, by default it is root
$pass = 'PASSWORD';//keep it blank if you have not given any password for your database else type your password here
// Connect to the database
$this->link = mysql_connect($host, $user, $pass);
mysql_select_db($db);
register_shutdown_function(array(&$this, 'close'));
}
//*** Function: query, Purpose: Execute a database query ***
function query($query) {
$this->theQuery = $query;
return mysql_query($query, $this->link);
}
//*** Function: fetchArray, Purpose: Get array of query results ***
function fetchArray($result) {
return mysql_fetch_array($result);
}
//*** Function: close, Purpose: Close the connection ***
function close() {
mysql_close($this->link);
}
}
?>
Step 6: Now, last step of code is to write your check.php file. It will search through all used usernames and give back the desired result.
<?php
//Followinh line will include dbConnector.php file to make connection with the database
include("dbConnector.php");
$connector = new DbConnector();
$username = trim(strtolower($_POST['username']));
$username = mysql_escape_string($username);
//below is the sql query to select username values from database
$query = "SELECT username FROM usernameCheck WHERE username = '$username' LIMIT 1";
$result = $connector->query($query);
$num = mysql_num_rows($result);
echo $num;
mysql_close();
?>
Step 7: The code above look for the username which is typed in username field in the database, if its found, then $num would be >= 1. It means that username already exist in database hence error indication will be displayed.
In this way, you can easily set up this validation measure in any of your registration form. Thank You!
Subscribe to:
Post Comments (Atom)
0 Responses to “Instant username validation using jQuery in PHP”
Post a Comment